@all
To continue Endianes problem, i need some help again for my lame knowledge :)
Is "bit-shifting" (<< and >>) are endian unaware in end ?
What i mean is i have for example that kind of code:
// A8R8G8B8
struct sVec4;
struct sCompressedVec4
{
u32 argb;
void setA8R8G8B8 ( u32 value )
{
argb = value;
}
void setColorf ( const video::SColorf & color )
{
argb = core::floor32 ( color.a * 255.f ) << 24 |
core::floor32 ( color.r * 255.f ) << 16 |
core::floor32 ( color.g * 255.f ) << 8 |
core::floor32 ( color.b * 255.f );
}
void setVec4 ( const sVec4 & v );
// f = a * t + b * ( 1 - t )
void interpolate(const sCompressedVec4& a, const sCompressedVec4& b, const f32 t)
{
argb = PixelBlend32 ( b.argb, a.argb, core::floor32 ( t * 256.f ) );
}
};
inline void sCompressedVec4::setVec4 ( const sVec4 & v )
{
argb = core::floor32 ( v.x * 255.f ) << 24 |
core::floor32 ( v.y * 255.f ) << 16 |
core::floor32 ( v.z * 255.f ) << 8 |
core::floor32 ( v.w * 255.f );
}
void setA8R8G8B8 ( u32 argb )
{
x = ( ( argb & 0xFF000000 ) >> 24 ) * ( 1.f / 255.f );
y = ( ( argb & 0x00FF0000 ) >> 16 ) * ( 1.f / 255.f );
z = ( ( argb & 0x0000FF00 ) >> 8 ) * ( 1.f / 255.f );
w = ( ( argb & 0x000000FF ) ) * ( 1.f / 255.f );
}
void setR8G8B8 ( u32 argb )
{
r = ( ( argb & 0x00FF0000 ) >> 16 ) * ( 1.f / 255.f );
g = ( ( argb & 0x0000FF00 ) >> 8 ) * ( 1.f / 255.f );
b = ( ( argb & 0x000000FF ) ) * ( 1.f / 255.f );
}
How it all should be done for PPC ? I mean all those << and >> seems like that all should be different for us.
Visually, problem with that code (well, i am not 100% sure that is _that_ code, but pretty possible, as only bit-shifting operations i find is those ones), looks like this:
On PPC (os4):
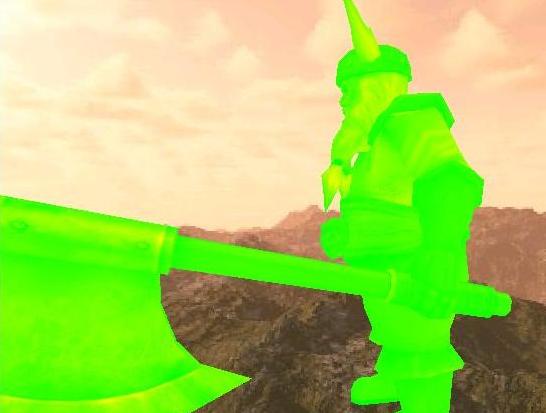
On X86 (win32)
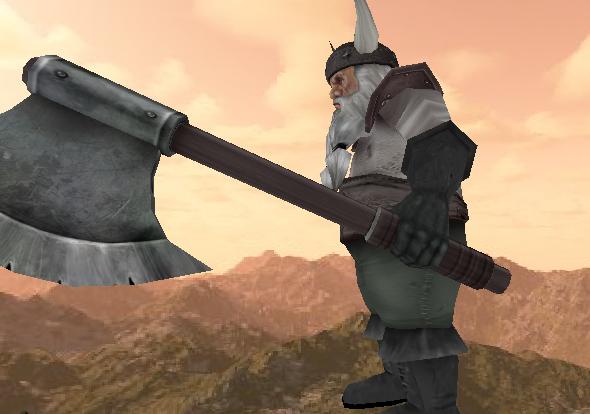
As can be seen "terrain" scene (earth and sky) looks fine, but colors of the textures on the dwarf looks different. That why i think about those functions i quote..
Whole file is here:
Vertex.h
Edited by kas1e on 2018/2/2 13:23:38